JavaScript SDK¶
The JavaScript SDK is intended for apps developers that integrate with the Waves Enterprise platform. Using JavaScript SDK, users of developed applications sign and send all types of transactions to the blockchain network.
The JavaScript SDK uses REST API methods to work with the blockchain. Unlike direct interaction with the blockchain through a traditional REST API tool an application signs the transaction locally in the browser or in the Node.js environment without addressing to the node. The signed transaction is sent directly to the network. This way of working with the blockchain is more convenient and effective for developing third-party services and applications that interact with the blockchain network. Data is transmitted and received in json format over the HTTPS Protocol.
The JavaScript SDK works both in the browser and in the Node.js environment. Web applications use the browser option, and backend applications use the Node.js environment operation. If you want to work without a browser, you will need to install the LTS version to use the JavaScript SDK Node.js. The JavaScript SDK package itself and instructions for installing and initializing it can be found in GitHub. The General scheme of the JavaScript SDK is shown below.
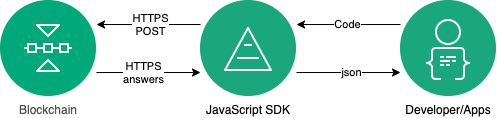
JavaScript SDK kit¶
JavaScript SDK includes basic tools for work, as well as two auxiliary tools:
transactions-factory - the component is intended for correct serialization into bytes of all types of transactions for subsequent signing with a private key.
signature-generator - a component that signs transactions and supports GOST and Wave cryptography.
Node cryptography methods in the JavaScript SDK¶
For realization of cryptographic algorithms, the SDK supports the cryptographic methods of the node REST API:
crypto/encryptCommon
crypto/encryptSeparate
crypto/decrypt
Authorization in the blockchain via the JavaScript SDK¶
An application user applies standard authorization tools in the Waves Enterprise blockchain. For more information about authorization methods, see authorization methods.
The JavaScript SDK supports authorization in the browser and in the Node.js environment. The Fetch API interface is used for authorization in the browser. For Node.js the Axios HTTP client is used.
Signing and sending transactions to the blockchain¶
An app can create, sign, and send any transaction to the blockchain. See transactions section to learn more about transactions.
The creation of a transaction is initiated by an app.
All transaction fields are serialized to bytes and signed with the user’s private key directly in the browser or in the Node.js environment.
The JavaScript SDK sends a transaction to the blockchain using the corresponding HTTP POST request.
An app receives a response in the form of a transaction hash to the executed HTTP POST request.
In order to obtain a transaction ID before its sending use a separate method:
const txHash = await Waves.API.Node.transactions.getTxId('transfer', txBody, { publicKey })
Creating a seed phrase¶
An app works with the seed phrase in the following ways:
Creates a phrase in a completely random order.
Creates an encrypted phrase with the specified password.
Decrypts the phrase using the specified password.